Welcome friends. In the React series. Today we will learn a new topic ‘Refs and the DOM’.
Table of Contents
Refs and the DOM – Introduction
In the ‘Refs and the DOM’ blog post, We will learn & understand the practical use of refs using DOM in React. We will also learn 4 types of creating and using Refs in React, important points by React documentation, advantages, and disadvantages.
Before using refs, props are similar to JS function arguments which are defined in HTML elements and passed through The standard approach for a parent component to interact with its child elements is through props.
E.g. – to modify the child, you need to re-render it with new props because props are immutable.
But sometimes we want to access DOM nodes or React elements that are created in the render method of components, Props aren’t exactly helpful in that scenario.
Refs and the DOM – Prerequisites
- Development Setup:
- Revise of React Concepts
Refs and the DOM – Terminologies
- Props: React Props means function arguments in JavaScript and attributes in HTML. They are arguments passed into React components. Props are passed to components via HTML attributes.
- Refs in React: Refs are used to giving for accessing DOM nodes or React elements created in the render method.
- DOM (Document Object Model):. The Document Object Model (DOM) is a programming API for defining the logical structure of documents and the way a document is accessed and manipulated.
Callback: A callback function is a function that is passed into another function as an argument, which is then invoked inside the outer function to complete some kind of routine or action.
What are Refs in React
“In simple terms, Refs refs give access to the underlying DOM element.”
As per react documentation, React ref Refs provide a way to access DOM nodes or React elements created in the render method.
While writing the React app, it is recommended to use the component-based architecture but there might be a situation where you may have to manage or imperatively change the DOM element. So to achieve, this React provides a new way called refs.
Refs and the DOM – When to use and don’t
Below are the points highlighted when to use Refs and when to not.
- Use Refs to DOM manage action similar text selection, element focus, or media playback.
- Triggering imperative animations.
- Integrating process with third-party DOM libraries.
Note: It is recommended to avoid using refs for other operations that can be done declaratively.
Ways of Creating and accessing DOM using Ref
1. Using Callback Refs
What is Callback Refs
In callback refs, we have to provide a callback function to ref props. So the function receives input as a DOM element which we can store in a variable for later use in the application.
For example: when a user clicks on the button the input element should have focus. First, I am creating a component along with input and a button.
I am dividing this into three steps.
- Create a callback function and bind this in the constructor
- Assign created our callback ref
- Create on the click handler function
- Create a callback function and bind this in the constructor
Example: Steps for creating callback refs
1. Create a callback function and bind this in the constructor
First, create a callback function and bind this to the constructor. I have created a callback function called inputElementRef.
Example:
import React, { Component } from "react";
class CallbackRefExample extends Component {
constructor(props) {
super(props);
this.inputElementRef = this.inputElementRef.bind(this);
}inputElementRef(inputElement) {
this.inputRef = inputElement;
}
render() {
return (
<div>
Callback Ref Example:
<br />
<input type="text" />
<button style={{ margin: "8px" }}>Click</button>
</div>
);
}
}
export default CallbackRefExample;
2. Assign created our callback ref
Assign the inputElementRef() function to ref props of an input element
<input type="text" ref={this.inputElementRef}/>
We have now created our callback ref.
3. Create on the click handler function
create on the click handler function to call the focus method using inputRef.
handleClick(){
this.inputRef.focus();
}
4. Assign this function to the onClick event of the button
<button class=”btn btn-success callback-ref”>Submit</button>
Final Code after merging all above steps:
import React, { Component } from "react";
class CallbackRefDemo extends Component {
constructor(props) {
super(props);
}render() {
return (
<div>
Callback Ref Example:
<br />
<input type="text" />
<button class=”btn btn-success”>Submit</button>
</div>
);
}
}
export default CallbackRefDemo;
Output: The final output will be displayed as below:
Here, when the user clicks on submit button, the input textbox will be highlighted as below:
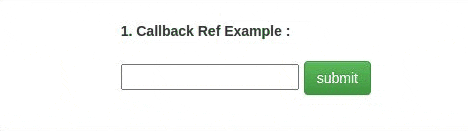
b. Using React.createRef() API
The react 16.3+ version of React has introduced a new API called ‘React.createRef()’ for creating refs. We do not need to create a callback function and assign it to ref props here.
Simply create a ref and store it into some variable and assign this variable to the ref prop of the DOM element.
As per the below example, we will create a functional component that has only one input element and one button.
Example:
import React, { Component } from "react";
export default function CallbackRefExample() {
return (
1. Callback Ref Example:
<br />
<input type="text" />
<button class=”btn btn-success callback-ref”>Submit</button>
</div>
);
}
Now create a variable called inputRef and assign it with React.createRef() API.
let inputRef = React.createRef();
Now apply this inputRef to ref props of the input element. After that, create an onClick handler for a button so that when the button onClick event fires, we have to focus on the input element.
Final Component:
So the final component looks like this:
import React, { Component } from "react";
export default function CallbackRefExample() {
let inputRef = React.createRef();const handleClick = () => {
inputRef.current.focus();
};
return (
<div>
1. Callback Ref Example:
<br />
<input type="text" ref={inputRef} />
<button class="btn btn-success callback-ref" onClick={handleClick}> Submit </button>
</div>
);
}
Here, we will receive the mounted instance of the component in its current property of ref. That’s why we have invoked the focus() function.
inputRef.current.focus();
Output The final output should look like this:
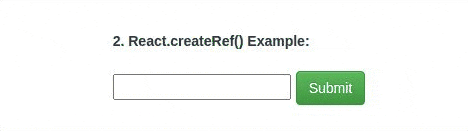
c. Using the useRef() hook
We can now create a ref using hooks. React provides us an inbuilt hook called useRef(). useRef hook is taking an initial value for the ref as input. Similar to React.createRef(), we have to create a variable that can store the ref reference for further use. Here is an example:
Example:
import React, { useRef } from "react";
export default function UseRefHookExample() { let inputRef = useRef(null);
const handleClick = () => { inputRef.current.focus(); };
return ( <div> <b> 3. useRef() hook Example:</b> <br /> <input type="text" ref={inputRef} /> <button class="btn btn-success use-ref" onClick={handleClick}> submit </button> </div> ); }
Output The final output should look like this:
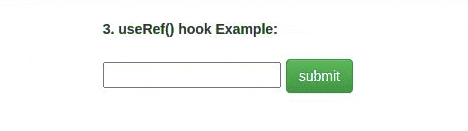
04. Using ref string (legacy)
In React, ref string is the old way of creating a ref.
The React team advises against using it, going so far as to label it as “legacy” in the documentation.
Example:
class App extends React.Component { state = { value: '' } handleSubmit = e => { e.preventDefault(); this.setState({ value: this.refs.textInput.value}) };
render() { return ( <div> <h1>React Ref - String Ref</h1> <h3>Value: {this.state.value}</h3> <form onSubmit={this.handleSubmit}> <input type="text" ref="textInput" /> <button>Submit</button> </form> </div> ); } }
ReactDOM.render(
, document.getElementById("root"));
Output:
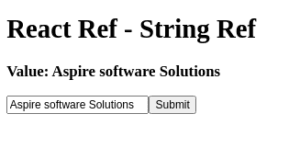
Important points for refs using DOM in react
Caveats (Warning) with callback refs
If the ref callback is defined as an inline function, it will get called twice during updates, first with null and then again with the DOM element.
This is because a new instance of the function is created with each render, so React needs to clear the old ref and set up the new one. You can avoid this by defining the ref callback as a bound method on the class, but note that it shouldn’t matter in most cases.
Let’s conclude the topic.
Conclusion
In this article, I have explained different ways to create Refs in React JS and also created simple examples using ref.
In the end, I hope that you enjoyed this article, and please do not hesitate to send us your feedback or comments. If you have any questions or need further assistance, feel free to Contact us . We're here to help!