Table of Contents
React Redux – Introduction
In the blog ‘react redux’, we will explore the understanding of the fundamental Redux concepts like what is react, the advantages and disadvantages of redux, redux core principles, redux in layman terms, and redux examples with explanations.
Terminologies
- State: State is a term from React it is an object that keeps data contained within a component. It decides how a component acts and renders.
- State management: State management is normally the management of the state of multiple user interface controls or components.
What is React Redux
Redux is a known lightweight state management tool for JavaScript applications. It is made by Dan Abramov and Andrew Clark and released in 2015.
Redux is the most in-demand state management solution. It helps you write apps that behave in the same, are easy to test, and can run the exact way in different environments (client, server, native). The important key factor is the entire application is handled by one state object is called React Store.
React Redux in Layman terms
Banking Example: Checking and Savings
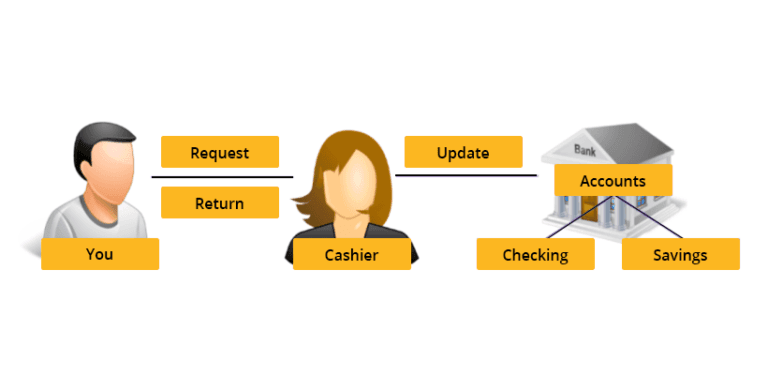
Imagine you visit a bank account and the bank provides checking and savings facilities. If you deposit or make transactions, it will reflect on your account balance. Here, The bank acts as the main store for all customer accounts including yours.
Let’s see terminologies of react-redux with banking example in a layman way
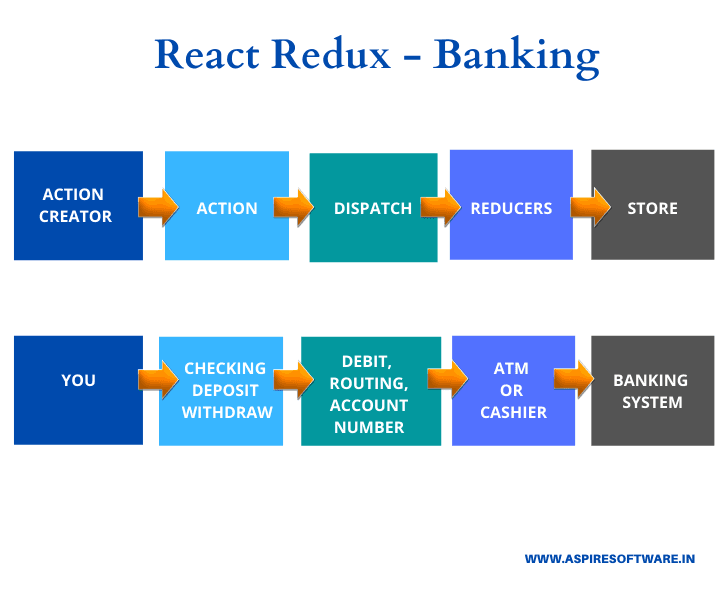
Store 🏛️ : Your banking service (Any bank like SBI, ICICI)
Action 💸: Your Action – WITHDRAW_MONEY or DEPOSIT_MONEY
Reducer 🏧👩💼: The Cashier or ATM that updates your account each time you visit the branch
Dispatch 💳: Your Debit, Routing, Account Number that works as deposit or withdrawal.
Redux’s Three core principles
According to Redux’s official documentation, Redux was established on three core principles:
- One Store for One App: The state of your whole application is stored in an object tree within a single redux store.
- The state must be Read-only: Make sure that the application state is read-only and requires changes to be performed by emitting a descriptive action.
- Use Pure Functions for State transfer To define how the state tree is transformed by actions, it is recommended to write pure reducer functions.
With the whole state of your application centralized in only one location, so each component has direct access to the state (at least without sending props to child components, or callback functions to parent components).
Main concepts of React Redux
Redux introduces actions, action creators, dispatch, reducers, and stores. Redux’s these concepts are used to create an easy state management architecture.
- Action
- Action Creators
- Dispatch
- Reducer
- Redux store
Action
Actions are simple and plain JavaScript objects that describe WHAT happened but don’t describe HOW the app state changes.
Technical: Actions are in the formation of Javascript objects, containing a type and an optional payload.
One important thing to remember is that Redux needs action objects to
contain a type field. This field is used to describe what type of actions
are dispatching. it should commonly be a constant that you export from a
file.
For example: In the below example, when the user clicks the “Add Note” button, we will dispatch something similar to the following action to our store. Below action contains properties first type and second content. Here type contains Actions and the content is optional.
{ type: ADD_NOTE,
title: 'Some Title',
content: 'This is an action object' }
Action creators:
Action creators are normal javascript functions that enable you to create actions. They are functions that return action objects, and next, the returned object is sent to different reducers in the application.
Example:
function addNote(title, content) {
return { type: ADD_NOTE, title: title, content: content };
}
Dispatch:
The dispatch () method is used to call actions anywhere in the app.
dispatch(addTodo(text));
Reducer:
Reducers are pure functions that explain HOW the app state changes.
Reducers are functions that take the current state and an action as arguments and return a new state result.
React Redux Example
import { createStore } from 'redux'
function count(state = 0, action) {
switch (action.type) {
case 'increase':
return state + 1
case 'decrease':
return state - 1
default:
return state
}
}
let store = createStore(counter)
store.subscribe(() => console.log(store.getState()))
store.dispatch({ type: 'increase' })
store.dispatch({ type: 'decrease' })
Explanation:
- Line 2 – 11: This implements a reducer which is known as a pure function with (state, action) => state signature. The function converts the initial state into the next state based on the action.type.
- Line 12: Creates a Redux store that holds the state of the app. Its API is { dispatch, subscribe, getState }. The createStore is a member of the Redux library.
- Line 13: subscribe() is applied to update the UI in response to state changes.
- Line 14 – 15: An action is dispatched to mutate the internal state.
Redux store
Redux store is an object, not a class. It contains the state of the application.
The Redux store is the application state which is stored as objects. Whenever the store is updated, it will update the subscribed React components that are subscribed to it. You need to create stores with Redux. The store has the ability of reading, storing and updating the state
We can pass middleware to the store to deal with the processing of data to keep a log of different actions that change the state of stores. All the actions return a new state through reducers.
Example:
import { createStore } from 'redux';
import reducers from '../reducers/reducers';
export default createStore(reducers);
Advantages and Limitations of Redux
- State transfer: State is stored in a single place called the ‘store.’ State also allows you to call state data from any component simply.
- Predictability: Redux is “a predictable state container for JavaScript apps.” Because reducers are pure functions, the equivalent result will always be produced when a state and action are passed in.
- Maintainability: Redux provides a strict structure for the code and state for better management. It makes the architecture easy to replicate and scale.
- Ease of testing and debugging: Redux makes it easy to test and debug code. It offers powerful tools Redux DevTools in which you can time travel to debug, track changes, and streamline your development process.
Conclusion
"When your website scales in size and your components begin making use of shared data, then yes, Redux will save you time rather than take it away.
When the state grows with too many properties, it can get difficult to keep up with what app interactions are changing your app’s state. Not only that, but if two components share the same state and don’t have a parent-child relationship, then you’d have to duplicate the state and update it in two places.
This is why Redux’s global store ability is effective and helpful for keeping things clean and maintainable.
Ready to streamline your development process with Redux? Get in touch with our team today!