Welcome friends, In the ‘The Complete Beginner’s Guide to Routing in React’ article we will explain all about routing in react, types of routing, examples of routing, and many more.
Table of Contents
Routing in React – Introduction
Routing is the feature in react to jump between several parts of an application when a user enters in the browser a URL or clicks an element (button, link, icon, image, etc) within the application.
Prerequisites
- Node and NPM
-
Basic React Knowledge of react
- Try React Introduction
- Invest 5 minute React JS
- Any Text Editor – Visual Studio Code
What is Routing in React
React Router is a very useful, standard library for routing in React. It offers the navigation among views of different components in a React Application, also allows changing the browser URL, and keeps the UI in sync with the URL.
Main Components of Routing in React:
The Main Components of React Router in Routing in React are:
- Link
-
Route
- Exact
- Path
- component
- switch
1. Link: The link component is used for creating links to different routes & implement navigation around the application. It works as an HTML anchor tag.
Use: The link component uses the ‘to’ prop to describe the location where the links should navigate to.
Example:
<div className="App">
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About Us</Link>
</li>
<li>
<Link to="/contact">Contact Us</Link>
</li>
</ul>
</div>
Now, run your application on the localhost and click on the links you created. You will notice here the URL changing according to the value into props of the Link component
2. Route: Route is known as the conditionally shown component which renders some UI when its path matches the given current URL.
Use: The route component is used to establish the link between the component’s UI & the URL. To include routes to the application, first, add the code given below to your file app.js.
Example:
<Route exact path='/' component={Home}></Route>
<Route exact path='/about' component={About}></Route>
<Route exact path='/contact' component={Contact}></Route>
Components are generally linked and clicking on any link will render the component linked with it.
Let us now try to explain the props associated with the Route component.
- exact:
exact in react-navigation attribute is used to match the exact value with the given URL.
For Eg., exact path=’/about’ will only render the component if it matches exactly the path but if we remove it from the syntax, then UI will still be rendered even if the structure is like /about/13.
- path:
Path in react-navigation specifies a pathname we assign to the component.
- component:
The component in react-navigation refers to the component which will render on matching the path.
Note: By default, routes are inclusive so more than one Route component can match the URL path & render at the same time. For single-component rendering, we should use the switch.
4. Switch:
The switch component is used to render only the first route which matches the location instead of rendering all matching routes.
Use: To render a single component, Bind all the routes inside the Switch Component. Switch groups together several routes, iterates over them, and finds the first one which matches the path. in that way, the corresponding component to the path is rendered.
Example:
<Switch>
<Route exact path='/' component={Home}></Route>
<Route exact path='/about' component={About}></Route>
<Route exact path='/contact' component={Contact}></Route>
</Switch>
Types of Routing in React
In Routing in React, React Router provides two different kinds of routes:
- MemoryRouter
- BrowserRouter
- HashRouter
MemoryRouter
What is MemoryRouter:
MemoryRouter keeps the URL changes in memory, not in the user browsers. It maintains the history of the URL in memory. It doesn’t alter the URL in your browser.
Memoryrouter doesn’t support forward and backward compatibility
MemoryRouter Syntax
import { MemoryRouter as Router } from 'react-router-dom';
MemoryRouter Use:
It is useful in testing and non-browser environments like React Native.
MemoryRouter Example:
import React, { Component } from 'react'; import { MemoryRouter as Router, Route, Link, Switch } from 'react-router-dom'; import Home from './component/home'; import About from './component/about'; import Contact from './component/contact'; import './App.css';
class App extends Component { render() { return ( <Router> <div className="App"> <Link to="/">Home</Link> <Link to="/about">About Us</Link> <Link to="/contact">Contact Us</Link>
<Switch> <Route exact path='/' component={Home}></Route> <Route exact path='/about' component={About}></Route> <Route exact path='/contact' component={Contact}></Route> </Switch> </div> </Router> ); }
} export default App;
2. BrowserRouter:
What is BrowserRouter:
The <BrowserRouter> is the more popular of the two because it uses the HTML5 History API to keep your UI in sync with the URL. BrowseRouter builds classic URLs.
BrowserRouter Syntax:
import { BrowserRouter as Router } from 'react-router-dom';
BrowserRouter Use:
It is used for handling the dynamic URL.
BrowserRouter Example:
import React, { Component } from 'react'; import { BrowserRouter as Router, Route, Link, Switch } from 'react-router-dom'; import Home from './component/home'; import About from './component/about'; import Contact from './component/contact'; import './App.css';
class App extends Component { render() { return ( <Router> <div className="App"> <Link to="/">Home</Link> <Link to="/about">About Us</Link> <Link to="/contact">Contact Us</Link>
<Switch> <Route exact path='/' component={Home}></Route> <Route exact path='/about' component={About}></Route> <Route exact path='/contact' component={Contact}></Route> </Switch> </div> </Router> ); }
} export default App;
https://application.com/dashboard /* BrowserRouter */
3. HashRouter:
What is HashRouter
<HashRouter> uses the hash portion of the URL (window.location.hash) to keep your UI in sync with the URL. HashRouter builds URLs with hash.
HashRouter Syntax
import { HashRouter as Router } from 'react-router-dom';
HashRouter Use:
HashRouter is used for handling the Static Request.
HashRouter Example:
import React, { Component } from 'react'; import { HashRouter as Router, Route, Switch, Link } from 'react-router-dom'; import Home from './component/home'; import About from './component/about'; import Contact from './component/contact'; import './App.css';
class App extends Component { render() { return ( <Router> <div className="App"> <Link to="/">Home</Link> <Link to="/about">About Us</Link> <Link to="/contact">Contact Us</Link>
<Switch> <Route exact path='/' component={Home}></Route> <Route exact path='/about' component={About}></Route> <Route exact path='/contact' component={Contact}></Route> </Switch> </div> </Router> ); }
} export default App;
https://application.com/#/dashboard /* HashRouter */
Note:
If you need to support legacy browsers that don’t support the History API, you should use <HashRouter>, Otherwise <BrowserRouter> is the better choice for most use cases.
Advantages Of React Router
The Advantages of React Router is given below:
- Using Routing, it is not compulsory to set the browser history manually.
- Link uses to navigate the internal links in the react application. It is alike to the anchor tag.
- It uses the Switch feature for rendering.
- The Router needs only a Single Child element.
- In Routing, every component is specified.
Example: How to create Routing in React
We will learn routing with the following steps:
Step 1: Create a React Application
Step 2: Install a React Router dependency
Step 3: Create Components:
Step 4: Add a Routes for component
Step 1: Create a React Application
First of a create react ‘aspire-router-example’ app through the below command:
npx create-react-app aspire-router-example
Step 2: Install a React Router dependency
A quick way to install the ‘react-router’ is to run the below code snippet in the terminal.
npm install react-router-dom
Step 3: Create Components:
In this step, we will create three components. The AppComponent will be a tab menu. The other three components are HOME, ABOUT, and CONTACT.
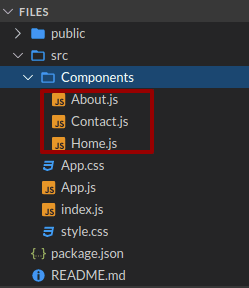
-> Home.js
import React from "react";
function Home() {
return (
<div>
<h1> Welcome to Homepage </h1>
<p>
Read react articles on
<a target="_blank" href="https://www.aspiresoftserv.com/blog">Aspire Blog </a>
</p>
</div>
);
}
export default Home;
-> About.js
import React from "react";
function About() {
return <h1> About us page </h1>;
}
export default About;
-> Contact.js
import React from "react";
function Contact() {
return <h1> Contact Page </h1>;
}
export default Contact;
Step 4: Add a Routes for component
Now, we will add routes to the app. Instead of rendering the App element like in the previous example, this time the Router will be rendered. Here we will set components for each route.
App.js
<Router>
------
<Route exact path="/" component={Home} />
<Route exact path="/about" component={About} />
<Route exact path="/contact" component={Contact} />
</Router>
When the app is started, we will see three clickable links which can be used to alter the route
App.js
import "./App.css"; import { BrowserRouter as Route, Router,, Link } from "react-router-dom"; import React from "react"; import Home from "./Components/Home"; import About from "./Components/About"; import Contact from "./Components/Contact";
function App() { return ( <div className="App"> <Router> <div className="App"> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About Us</Link> </li> <li> <Link to="/contact">Contact Us</Link> </li> </ul> </div>
<Route exact path="/" component={Home} /> <Route exact path="/about" component={About} /> <Route exact path="/contact" component={Contact} /> </Router> </div>
); } export default App;
Output:
Working Example:
https://stackblitz.com/edit/react-jwkhuw?embed=1&file=src/App.js
Let’s conclude,
Conclusion:
As we have seen what is Routing, types of Routing in React, and many more. React components are the basic building blocks in React. From above, We went through topics like React Component, React Lifecycle, Types of React Components, Functional Component, Class Component and, frequently asked questions on React Components.
Ready to dive deeper into the world of React components? Explore the complete series in the next section and feel free to reach out with any questions. Contact us to continue your React journey!