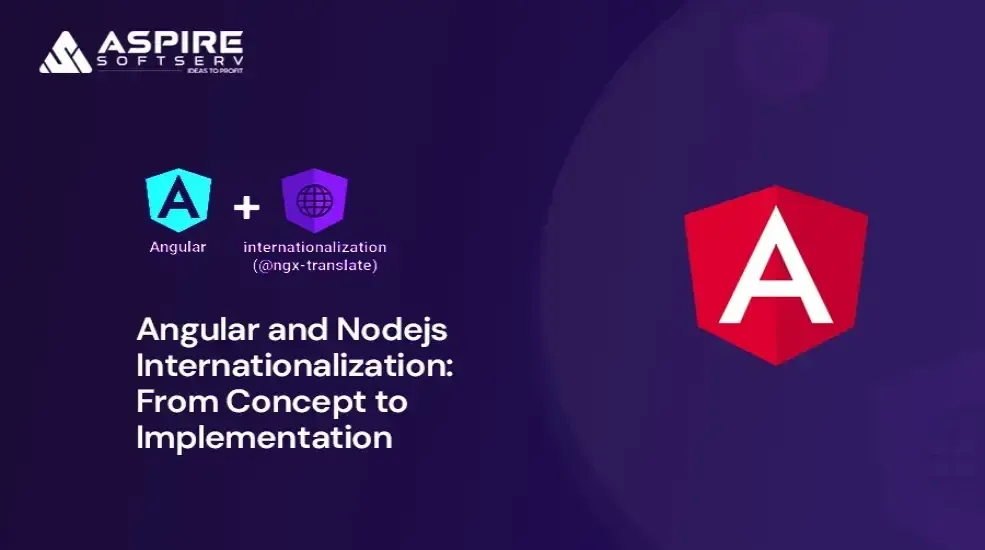
As we can see people are looking for the businesses who knows how to work in an internationalization way, which means you have to create software that will be understood by everyone, from any part of the world.
There are many businesses in the market but only a few people are capable of providing the best software services to their clients. The demand is very high and only a few companies can provide the best node.js developers or angular developers to the clients for immense results. To know more about internationalization read till the end.
What is Angular?
Angular is an open-source, JavaScript framework written in TypeScript, managed by Google. Its primary purpose is to develop single-page applications. As a framework, Angular has clear advantages while also providing a standard structure for Angular developers to work with, enabling them to create large applications in a maintainable manner.
What is Internationalization?
Why Do We Use Internationalization?
We use internationalization to make software applications accessible and usable by people from different languages and cultural backgrounds. By enabling the translation of text and adapting formats to regional conventions, internationalization ensures a better user experience for global audiences.
Advantages of Internationalization:
Internationalization helps companies achieve growth, improve their profitability, spread the risk, and increase competitiveness.
Improved User Experience: By providing content in users' preferred languages and adapting formats to regional conventions, internationalization enhances the user experience, making the software more accessible and user-friendly.
Illustrating Front-End Internationalization in Angular:
Follow the step-by-step solution to Implement this feature:
Prerequisites:
Step 1: - Installation
For Windows Users:
Install Chocolatey (if not already installed):
Install Node.js and npm:
- choco install nodejs
Install Angular
- npm install -g @angular/cli
For Ubuntu Users:
First run Command
- sudo apt update
Install Node.js and npm:
- sudo apt install nodejs npm
Install Angular
- npm install -g @angular/cli
For Mac Users:
Install homebrew (if not installed)
Homebrew is a package manager for macOS (and Linux, too). It is one of the first tools you'll need to set up a local development environment for programming on a Mac. Use it to install (and remove) software programs for the terminal, or command line.
Install Node.js and npm:
- brew install node
Install Angular
- npm install -g @angular/cli
Install nvm
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
Step 2: - Create an Angular Application:
- ng new internationalization
Step 3: - Installation of necessary packages:
- ng add @angular/localize
- npm i @ngx-translate/core
- npm i @ngx-translate/http-loader
Create one folder for i18n:
Create languages file.
fr.json
{
"greeting": "Bonjour, le monde!"
}
en.json
{
"greeting": "Hello world"
}
Add i18n configurations in angular.json.
"i18n": {
"sourcelocal":"en",
"locale":{
"fr":"src/assets/i18n/fr.json",
"en":"src/assets/i18n/en.json"
}
},
Source locale: The locale you use within the app source code ( en by default)
Locales: A map of locale identifiers to translation files.
import { AppComponent } from "./app.component.ts";
import { AppRoutingModule } from "./app-routing.module";
import { HttpClient, HttpClientModule } from "@angular/common/http";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { TranslateLoader, TranslateModule } from "@ngx-translate/core";
export function HttploaderFactory(http: HttpClient) {
return new TranslatehttpLoader(http, './assets/i18n/', '.json');
}
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
TranslateModule.forRoot({
loader: {
provide: TranslateLoader,
useFactory: HttploaderFactory,
deps: [[HttpClient]]
}
})
],
providers: []
})
TranslateLoader: Abstract class in ngx-translate library for loading translation data dynamically from different sources.
TranslateModule: Angular module provided by ngx-translate for integrating translation features into Angular applications.
TranslateHttpLoader: Implementation of TranslateLoader interface for asynchronously loading translation files over HTTP using Angular's HttpClient.
HttpClientModule and HttpClient: Description: Angular module and service for making HTTP requests to remote servers or APIs and handling response data.
TranslateModule.forRoot(): Initializes the translation module for the root Angular application.
Loader: Specifies the loader configuration for loading translation files.
Provide: Indicates the translation loader service to use.
UseFactory: Specifies a factory function to create the translation loader service.
Deps: Defines the dependencies required by the factory function, in this case, the HttpClient service.
This function HttpLoaderFactory is an Angular factory function used to create an instance of TranslateHttpLoader. It takes an HttpClient instance as a parameter and returns a new TranslateHttpLoader configured to load translation files from the specified path (./assets/i18n) with a JSON file extension.
App.component.ts
import { Component } from '@angular/core';
import { TranslateService } from '@ngx-translate/core'
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrl: './app.component.scss'
})
export class AppComponent {
constructor(public translateService:TranslateService){
const userlang = navigator.languages || "en";
const languagecode = userlang.split('-')[0];
this.translateService.setDefaultLang(languagecode);
this.translateService.use(languagecode);
}
}
Step 4: Add a Package Using npm
- npm install @ngx-translate/core
In the above image, we import the package translateservice and inject into the constructor In JavaScript, navigator.language is a property of the navigator object that represents the preferred language of the user's browser. It returns a string representing the language identifier (e.g., "en", "en-US", "fr") based on the browser's settings. This property is useful for implementing internationalization features in web applications, allowing developers to tailor the content and user interface based on the user's preferred language.
To change the preferred language settings, go to-> language -> Add language -> Add
If you want to translate your words into your native language, then add language in the Preferred Languages section.
You can see the output in French also in below image:
Illustrating Back-End Internationalization in Nodejs:
What is Nodejs?
A NodeJS is the framework that provides a runtime environment for server-side programming. It is an open-source platform, a cross-platform JavaScript runtime environment that runs web applications outside the client browser.
Step 1: - Create Nodejs App
- npm init –y
Step 2: -Install some packages using npm
- npm install i18next i18next-http-backend express
i18next: A comprehensive internationalization framework for JavaScript that facilitates multilingual support in web applications.
i18next-http-backend: A plugin for i18next that enables loading translations from HTTP backends, facilitating dynamic fetching of language resources.
Express: A fast, unopinionated, minimalist web framework for Node.js, providing a robust set of features for building web applications and APIs.
Create One Directory Structure and Create a Translation File:
en.json
{
"welcome": "Welcome to our app!",
"greeting": "Hello, {{name}}!"
}
fr.json:
{
"welcome": "Bienvenue dans notre application!",
"greeting": "Bonjour, {{name}}!"
}
server.js file
const express = require('express');
const translate = require('./translation');
const app = express();
// Configure express-session middleware
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true
}));
// Option 1: Translate based on a hardcoded language preference
app.get('/api/message', (req, res) => {
// Translate 'welcome' key to French ('fr')
const message = translate('welcome', {}, 'fr');
res.json({ message });
});
// Option 2: Translate based on the user's session language preference
// API endpoint
app.get('/api/message', (req, res) => {
// Translate 'welcome' key based on the user's session language preference
const message = translate('welcome', {}, req);
res.json({ message });
});
// Endpoint to set user's language preference
app.get('/set-language/:lang', (req, res) => {
req.session.lang = req.params.lang;
res.send('Language preference set successfully');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In a server.js file, we import the Express and translation files. We create an API that translates the message into French, as seen in the translate function in option 1, and another way to handle user preference language, as seen in the id option 2. If we have a hardcoded language preference, then we choose option 1; otherwise, we choose option 2.
transition.js
const i18next = require('i18next');
const i18nextMiddleware = require('i18next-express-middleware');
const session = require('express-session');
// Define your translations
const translations = {
en: require('./locales/en.json'),
fr: require('./locales/fr.json'),
// Add more languages here if needed
};
// Configure i18next with translations
i18next
.use(i18nextMiddleware.LanguageDetector)
.init({
fallbackLng: 'en',
resources: translations
});
// Middleware to handle translation
function translate(key, options = {}, req) {
const lang = req.session.lang || 'en'; // Retrieve language from session or default to 'en'
return i18next.t(key, options);
}
module.exports = translate;
fallbackLng: 'en': Specifies the default language to use if the desired language translation is not available. In this case, it's set to English ('en').
i18next.t (key, options) function is the core method for retrieving translated strings from your i18next internationalization library. It enables you to display content in the appropriate language for your users.
In our project, we have numerous JavaScript files. To translate static messages in any language, we just need to import the translation.js and translate the messages accordingly. In different projects, when just we need to translate certain static text in any language, we just need to import the translation file.
Conclusion:
Internationalization is the process of designing and developing a software application that can be adapted to different languages and cultural conventions without any need for significant modification. A Software Development company plays a crucial role in internationalization, as it involves making software accessible and usable by people around the world, regardless of their language or cultural background.
This entails creating applications that can support different languages and other regional preferences. By implementing internationalization, developers ensure that their software can reach a global customer base, fostering inclusivity and improving user experience across different cultures and regions.
Go Global with Internationalization! Take Your Business to New Heights