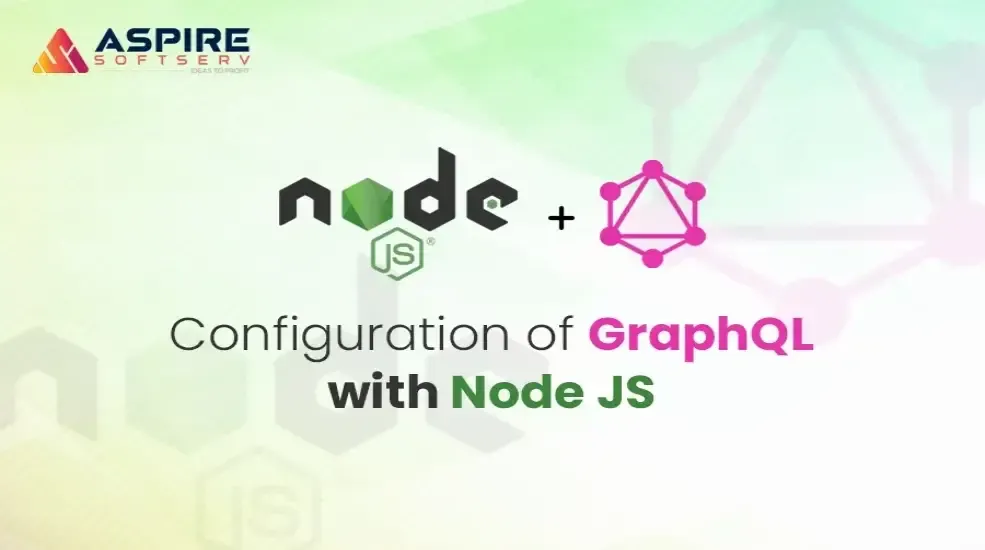
Introduction
Are you tired of the poor performance of REST APIs? Do you desire flexible data fetching and efficient searching? If so, go into the intriguing realm of GraphQL nodejs! This innovative query language allows you to request the exact information you need at the exact time. And how better to integrate GraphQL with Node.js framework?
It is essential, nevertheless, that you understand the settings before embarking on this exciting journey. Don't worry, though! This blog series will guide you through the configuring process step-by-step, serving as a handy compass.
Node.Js
Node.js is an asynchronous javascript runtime environment. With the help of it, you can create communication between the front-end and back-end.
There are 2 Types of API Functions:
Blocking Functions- This prevents any further operations until the current ones have finished, as demonstrated in the context of a REST API.
Non-blocking Functions- It continues executing the program without impeding other operations, exemplified by GraphQL.
GraphQL
GraphQL is a query language that produces data as a tree structure for your APIs (Application Programming Interface). It facilitates declarative data fetching, allowing users to specify precisely the required data from an API.
REST API
A call is made from a client to a server, and data is received back over the HTTP protocol. It is the simplest way to exchange information securely over the Internet.
There are primarily four different methods:
- GET: Used for reading data
- POST: Employed to create data
- PUT: Utilized to update data
- DELETE: Used for deleting data
Difference Between GraphQL and REST API:
The primary difference lies in the method through which data is sent from the server to the client using the API endpoint.
- REST API can be organized in terms of endpoints, whereas GraphQL can be arranged in terms of a schema.
- In REST API, the client sends an HTTP request for data and receives it as an HTTP response, whereas in GraphQL, the client requests data with queries.
- REST APIs adhere to a server-driven architecture, while GraphQL adopts a client-driven approach.
Why GraphQL?
- Good for large and complex data
- Reduced number of endpoints
- Fast and specific data retrieval
- Minimizing data and bandwidth wastage
- Examine the complete schema structure with real-time validation and updates.
See Also: Node JS Installation in 2023
Various Steps to Configure Graphql With Node.js:
To configure GraphQL, it is necessary to install Node.js in your system.
Step 1:
Create a directory for your project and open it in your preferred code editor.
Step 2:
Create two subdirectories within the project folder: one for the client side and another for the server side.
Step 3:
Navigate to the server-side directory in your terminal and proceed to install GraphQL and Apollo server within it. Using the following command:
- npm init
- npm install @apollo/server graphql
Apollo server is a library for managing data with GraphQL.
Install Express along with two additional dependencies, namely body-parser and cors, to facilitate communication between the client and server.
- npm install express body-parser cors
Step 4:
Create a file named 'index.js' in the server directory, and begin building your server using the following code:
const express = require("express"); const { ApolloServer } = require("@apollo/server"); const bodyParser = require("body-parser"); const cors = require("cors"); const startServer = async() => { const app = express(); const server = new ApolloServer({ typeDefs: {}, resolvers: {} }); app.use(bodyParser.json()); app.use(cors()); await server.start();
app.listen(5000, () => console.log("Server Started at PORT 5000")); } startServer();
In the code above, it is essential to include GraphQL configuration to inform the GraphQL server about the types of operations you intend to perform.
There are three types of operations:
Queries: Retrieve data
Mutations: Modify data
Subscriptions: Listen for live-streaming data
Example: In Express, you construct routes, while in GraphQL, you build a schema using type definitions.
Express(): It offers a wide range of functionalities for web and mobile application development, catering to creating single-page, multi-page, and hybrid web applications.
Cors(): With the assistance of CORS, we aim to facilitate communication between two distinct ports.
Step 5:
Define your schema in typeDefs, always represented as a string.
const server = new ApolloServer({ typeDefs: ` type Greeting { morningGreet: String } type Query { getGreeting: “”, sum(a: Int!, b: Int!): Int, getRandomNumber(min: Int!, max: Int!): Int
} `, resolvers: {}
});
The sum field is defined with arguments a and b, both of which are marked with ! meaning they are required and must be provided when calling the sum query.
The getRandomNumber takes two arguments min and max, both of type Int, and returns a random integer between min and max.
If you want to fetch data from your GraphQL server, then you use a query, and if you want to insert new data or modify existing data, then you use mutations in GraphQL.
Step 6:
Develop a resolver by adding logical stuff.
const server = new ApolloServer({
typeDefs: {},
resolvers: {
Query: {
getGreeting: () => “Rise and Shine.”,
sum: (a, b) => a + b,
getRandomNumber: (min, max) => {
return Math.floor(Math.random()*(max - min + 1))+ min;
}
}
}
});
GraphQL server supports three queries:
- GetGreeting
- Sum
- GetRandomNumber : Implemented the logic using JavaScript's Math.random() function.
Each has its own resolver function.
const express = require("express"); const { ApolloServer } = require("@apollo/server"); const bodyParser = require("body-parser"); const cors = require("cors"); const startServer = async() => { const app = express(); const server = new ApolloServer({ typeDefs:
type Greeting { morningGreet: String } type Query { getGreeting: “”, sum(a: Int!, b: Int!): Int, getRandomNumber(min: Int!, max: Int!): Int }
,resolvers: { Query: { getGreeting: () => “Rise and Shine.”, sum: (a, b) => a + b, getRandomNumber: (min, max) => { return Math.floor(Math.random()*(max - min + 1))+ min; } } }
}); app.use(bodyParser.json()); app.use(cors()); await server.start(); app.listen(5000, () => console.log("Server Started at PORT 5000")); } startServer();
Step 7:
Now, you are ready to run your server using the following command:
- node server/index.js
Step 8:
If the server runs successfully, proceed to make a query on the GraphQL playground to verify the retrieval of data.
GraphQL Playground:
GraphQL Playground functions as both a GraphQL Integrated Development Environment (IDE) and a query explorer, serving as a tool for constructing and validating queries.
GraphQL Playground offers a comprehensive and user-friendly environment for exploring, testing, and debugging GraphQL APIs, making it an essential tool for developers working with GraphQL.
Congratulations!! You have successfully built your GraphQL server.
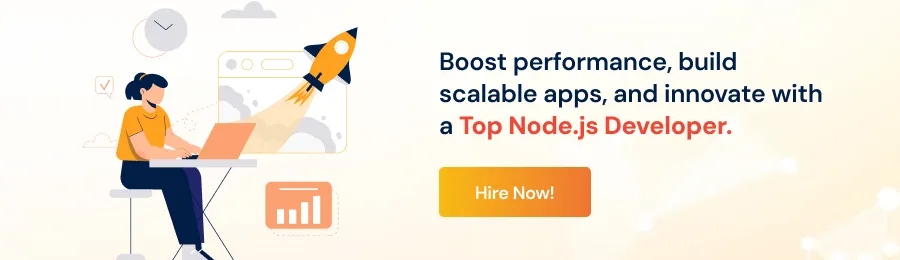
Conclusion
Congratulations on completing this introductory journey into the world of configuring GraphQL with Node.js! By now, you've gained a solid understanding of the fundamental concepts and steps involved in setting up a GraphQL server in a Node.js environment. Happy coding!
Looking for Help?
Seeking assistance with Node.js projects? Look no further! At Aspire SoftServ, we excel in offering expert help for all your Node.js development needs. Whether you require scalable web applications, real-time APIs, or backend system enhancements, our experienced team of Node.js developers is ready to assist. Hire Node.js developer today and ensure the success of your endeavors with us.